Exporting Visualizations and data#
Sometimes we want to save checkpoints from our data. That means we need to save the data in an appropriate format. The same goes for visualizations that can be used in presentations, reports, and designs.
Exporting data and visualizations are essential in many fields, such as data analysis, machine learning, and visualization design.
It involves converting data and visualizations into a format easily shared and used by others. Data export can be crucial when we need to save the state of our analysis or share it with colleagues or clients. Visualizations, on the other hand, can help communicate insights more effectively. Still, they must also be exported in a format that retains their quality and allows them to be easily inserted into presentations or documents.
In this context, understanding how to export data and visualizations is vital for anyone who works with data or creates visualizations.
How To#
import pandas as pd
import matplotlib.pyplot as plt
df = pd.read_csv("data/housing.csv")
df.head()
longitude | latitude | housing_median_age | total_rooms | total_bedrooms | population | households | median_income | median_house_value | ocean_proximity | |
---|---|---|---|---|---|---|---|---|---|---|
0 | -122.23 | 37.88 | 41.0 | 880.0 | 129.0 | 322.0 | 126.0 | 8.3252 | 452600.0 | NEAR BAY |
1 | -122.22 | 37.86 | 21.0 | 7099.0 | 1106.0 | 2401.0 | 1138.0 | 8.3014 | 358500.0 | NEAR BAY |
2 | -122.24 | 37.85 | 52.0 | 1467.0 | 190.0 | 496.0 | 177.0 | 7.2574 | 352100.0 | NEAR BAY |
3 | -122.25 | 37.85 | 52.0 | 1274.0 | 235.0 | 558.0 | 219.0 | 5.6431 | 341300.0 | NEAR BAY |
4 | -122.25 | 37.85 | 52.0 | 1627.0 | 280.0 | 565.0 | 259.0 | 3.8462 | 342200.0 | NEAR BAY |
df = df.join(pd.get_dummies(df.ocean_proximity))
df.to_csv("out.csv", na_rep="nan")
Writing any kind of file#
with open("out.txt", mode="w") as f:
f.write(df.to_string())
with open("out.txt", mode="w") as f:
f.write("Anything really")
with open("out.txt", mode="w") as f:
f.write(df.to_string())
with open("out.txt", mode="a") as f:
f.write("Anything really")
Saving Figures#
plt.plot([0,1,3,5])
plt.tight_layout()
plt.savefig("random.jpg", facecolor="k")
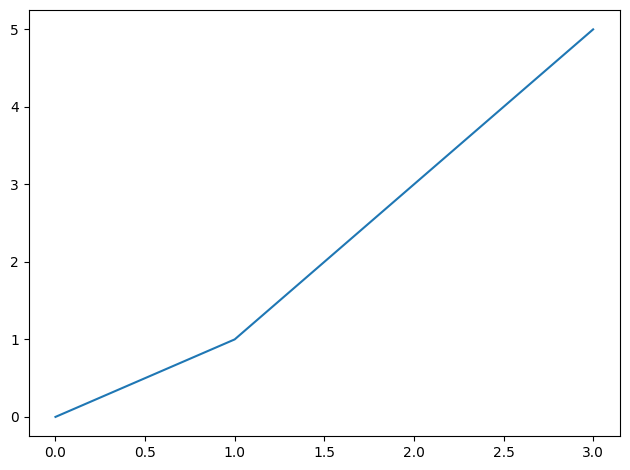